Object-Oriented Programming OOP in Python 3
Table Of Content
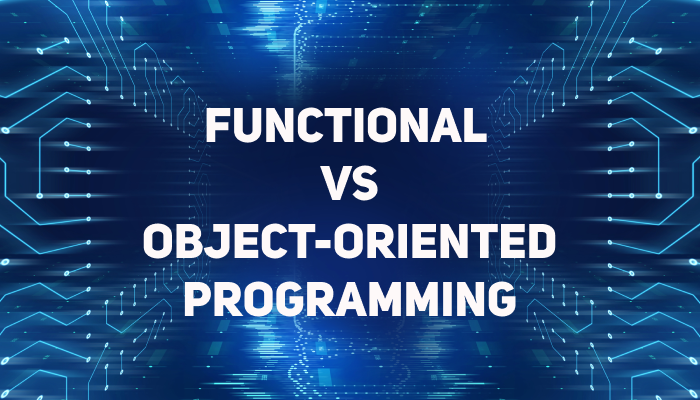
Segregation means keeping things separated, and the Interface Segregation Principle is about separating the interfaces. Therefore, when a class does not obey this principle, it leads to some nasty bugs that are hard to detect. Now that we have covered the basics of the principle, let's apply it to our Invoice application. The Open-Closed Principle requires that classes should be open for extension and closed to modification.
Template Method Pattern
This code demonstrates the creation of an Iron Man class with name and suit color attributes, as well as fly() and shootLaser() methods. For example, in a superhero class like Iron Man, attributes could be name and suit color, while methods might be fly() and shootLaser(). We can then assign values to the properties defined in the class to describe my bicycle without impacting other objects or the class blueprint itself. This is a much better approach, but a pitfall to watch out for is when type-hinting these interfaces. Instead of using a ShapeInterface or a ThreeDimensionalShapeInterface, you can create another interface, maybe ManageShapeInterface, and implement it on both the flat and three-dimensional shapes. The AreaCalculator class would handle all of the logic, violating the single responsibility principle.
Polymorphism in Object-Oriented Programming
The above noted principles form the foundation for the OOA approach. Notice that isinstance() takes two arguments, an object and a class. In the example above, isinstance() checks if miles is an instance of the Dog class and returns True.
Software Architecture
Instead, the updateArmorStrength() method is used to modify _armorStrength. In select learning programs, you can apply for financial aid or a scholarship if you can’t afford the enrollment fee. If fin aid or scholarship is available for your learning program selection, you’ll find a link to apply on the description page. That way, if we want to change the rules about studying archery, we only have to update the Student class, and all the code using it will still work. It would be helpful if we could represent the fact that students and professors share some properties, or more accurately, the fact that on some level, they are the same kind of thing. Projects that adhere to these principles can be shared with collaborators, extended, modified, tested, and refactored with fewer complications.
Object-Oriented Programming and the magic of Test-Driven Development - Towards Data Science
Object-Oriented Programming and the magic of Test-Driven Development.
Posted: Mon, 26 Aug 2019 07:00:00 GMT [source]
Subtype polymorphism as enforced by the type checker in OOP languages (with mutable objects) cannot guarantee behavioral subtyping in any context. Behavioral subtyping is undecidable in general, so it cannot be implemented by a program (compiler). Class or object hierarchies must be carefully designed, considering possible incorrect uses that cannot be detected syntactically. More recently, some languages have emerged that are primarily object-oriented, but that are also compatible with procedural methodology.

To keep the example small in scope, you’ll just use name and age. While the class is the blueprint, an instance is an object that’s built from a class and contains real data. It’s an actual dog with a name, like Miles, who’s four years old. In this tutorial, you’ll create a Dog class that stores some information about the characteristics and behaviors that an individual dog can have. The Open-Closed Principle states that classes should be open for extension but closed for modification. “Closed for modification” means that once you have developed a class you should never modify it, except to correct bugs.
YAGNI – You Ain’t Gonna Need It Principle
The Visitor Pattern is a behavioral design pattern in object-oriented programming that allows you to add further operations to objects without having to modify them. It i used when you have a set of objects with different types, and you want to perfrom a common operation on them without modifying their classes. The pattern achieves this by defining a separate visitor class or interface, which is responsible for performing operations on these objecs. Each object accepts the visitor and delegates the operation to it. The Adapter Pattern is a structural design pattern in object-oriented programming that allows objects with incompatilbe interfaces to work together. It acts as a bridge between two incompatible interfaces, making them compatible without changing acutal classes.
In the output above, you can see that you now have a new Dog object at 0x106702d30. This funny-looking string of letters and numbers is a memory address that indicates where Python stores the Dog object in your computer’s memory. An object contains data, like the raw or preprocessed materials at each step on an assembly line. In addition, the object contains behavior, like the action that each assembly line component performs. At each step of the assembly line, a system component processes some material, ultimately transforming raw material into a finished product.
How Do You Instantiate a Class in Python?
Attributes and methods can be set to private, preventing access from outside the class. Public methods and properties are used to access or update data, allowing the developer to control what information is visible. In this example, we create a "Superhero" class that acts as an interface. We then create IronMan and CaptainAmerica classes that extend the Superhero class and implement the fight() method. Finally, we create a startBattle() function that accepts any object that implements the Superhero "interface" and calls the fight() method.
This means that regardless of how many times the class is instantiated, there will always be only one instance, which can be accessed from any part of the program. You use inheritance in OOP to classify the objects in your programs per common characteristics and performance. This makes working with the objects and programming easier, because it enables you to mix general characteristics into a parent object and inherit these characteristics within the child objects. The concepts of OOP started to surface back in the 60s with a programming language called Simula. Even though back in the day, developers didn't completely embrace the first advances in OOP languages, the methodologies continued to evolve.
Finding things that are similar in your code and providing a generic function or object to serve multiple places/with multiple concerns. If you had to understand every single function in a big codebase you would never code anything. In this example, the Subject class notifies all registered observers when its notify method is called.
Comments
Post a Comment